Define and read environment properties
Let us see how to define environment specific properties and read them from code
Define Environment specific property files
Create JSON files to store properties specific to environment.
Let us create JSON files as below :
data_sets/QA/properties.json
: This file to hold environment properties for QA environmentdata_sets/UAT/properties.json
: This file to hold environment properties for UAT environment
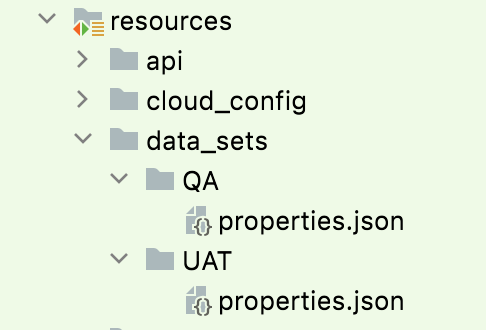
Add properties
data_sets/QA/properties.json
{
"property1": "property1_value_qa",
"property2": "property2_value_qa"
}
data_sets/UAT/properties.json
{
"property1": "property1_value_uat",
"property2": "property2_value_uat"
}
Config changes
In the previous article we have made config changes in qa.properties
and uat.properties
This is how properties would be read as:
Environment | Property | Value | |
---|---|---|---|
uat | property1 | property1_value_uat | |
uat | property2 | property2_value_uat | |
qa | property1 | property1_value_qa | |
qa | property2 | property2_value_qa |
Reading properties
- Inject DataSetsClient object
@Inject private DataSetsClient dataSetsClient;
- Read value of property
String property1 = dataSetsClient.getValue("property1", String.class);
Full Code
package ekam.example.web;
import com.google.inject.Inject;
import com.testvagrant.ekam.commons.data.DataSetsClient;
import com.testvagrant.ekam.testBases.testng.WebTest;
import org.testng.annotations.Test;
public class DataSetsClientTest extends WebTest {
@Inject private DataSetsClient dataSetsClient;
@Test(groups = "web")
public void shouldTestSomething() {
String property1 = dataSetsClient.getValue("property1", String.class);
System.out.println(property1);
String property2 = dataSetsClient.getValue("property2", String.class);
System.out.println(property2);
}
}
Execute tests
Execute tests against uat:./gradlew clean runWebTests -Dconfig=uat
The output would be:
property1_value_uat
property2_value_uat
Execute test against qa:./gradlew clean runWebTests -Dconfig=qa
The output would be:
property1_value_qa
property2_value_qa