Define Model for Test Data
Let us see how to define test data for environments and read them through code
Define Test data specific to environments
As an example, let us say we have an address that needs to be stored.
qa
Let us add data_sets/QA/address.json
as below
{
"address": [
{
"firstName": "Hari",
"city": "Bangalore",
"pinCode": "11001"
}
]
}
uat
Let us add data_sets/UAT/address.json
as below
"address": [
{
"firstName": "Puja",
"city": "Delhi",
"pinCode": "22002"
}
]
}
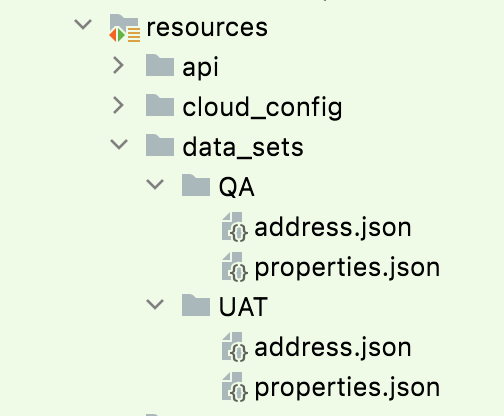
Model & DataClients
Now we have defined the test data as JSON, next steps are :
- Generate a Model to hold the JSON as an object
- Generate a DataClient which reads the Model
Create a folder called src/test/java/dataclients/address
to store Model
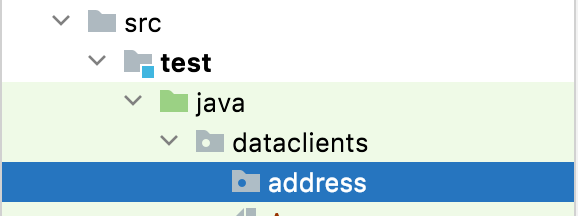
Generate Model
Copy json string representing address from address.json
to clipboard. Please copy only the object as shown below.
{
"firstName": "Hari",
"city": "Bangalore",
"pinCode": "11001"
}
Right-click on dataclients/address
folder → New → Generate POJO from JSON. Below window would open
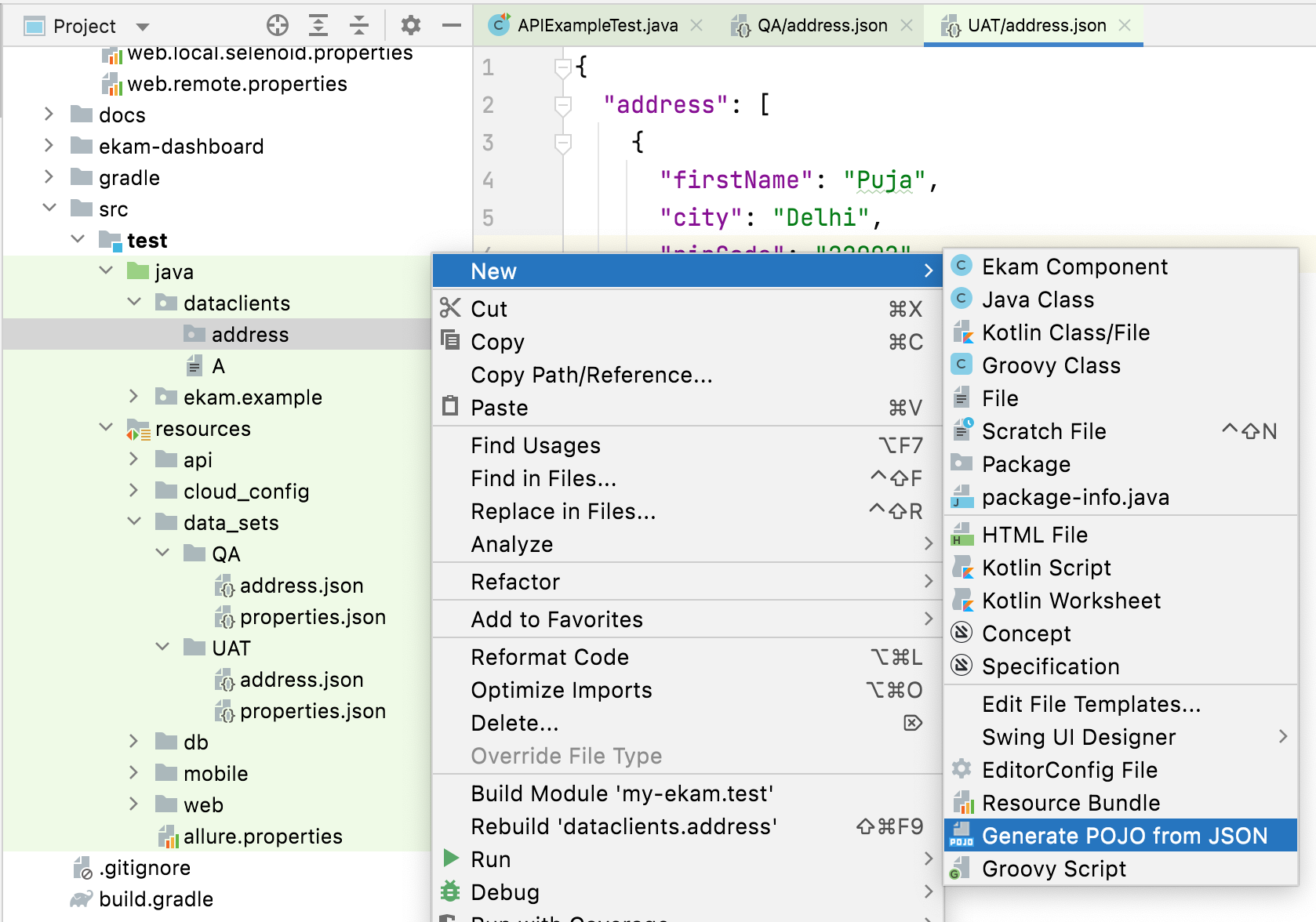
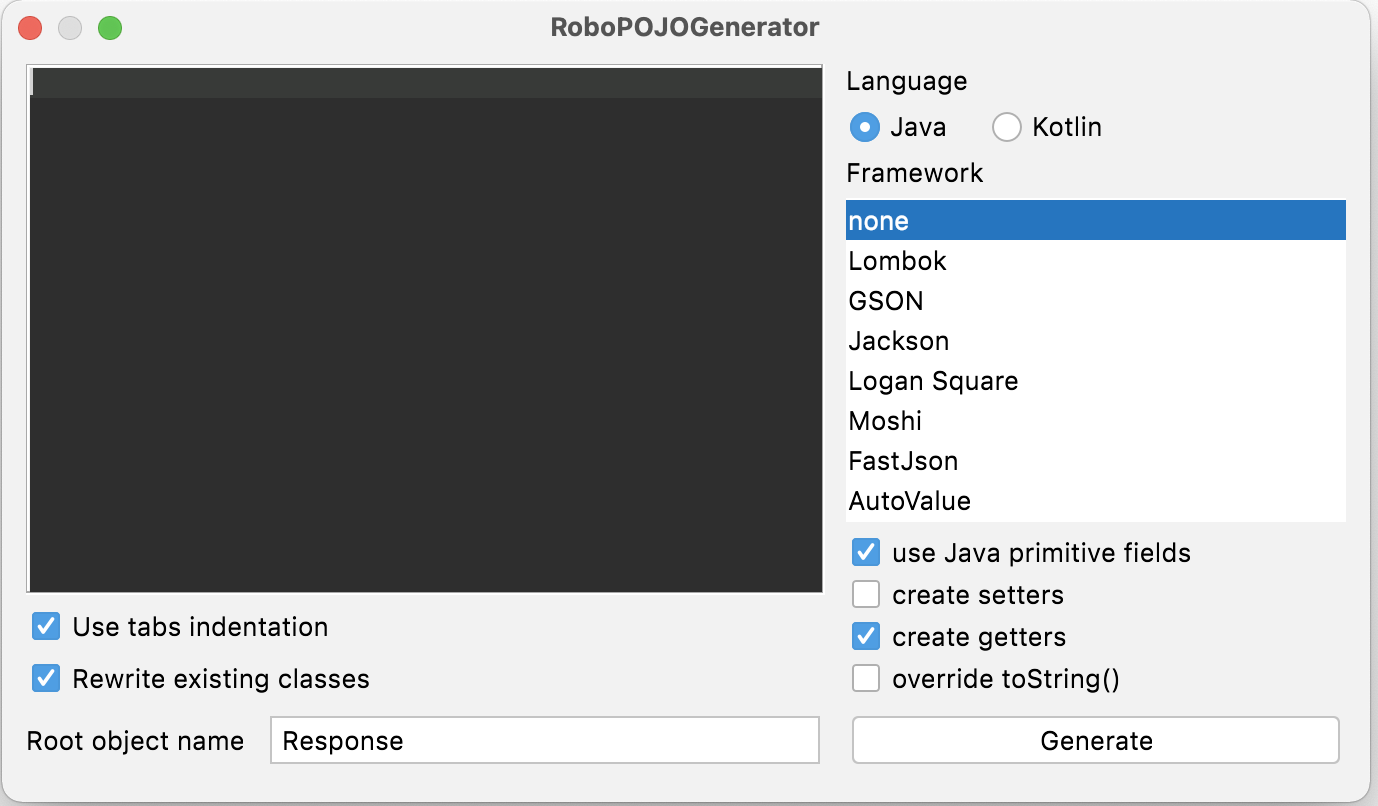
Paste the JSON from the clipboard. Enter Root object name is Address. Choose Lombok as Framework.
Click Generate button. This will Generate a class called Address with the below details.
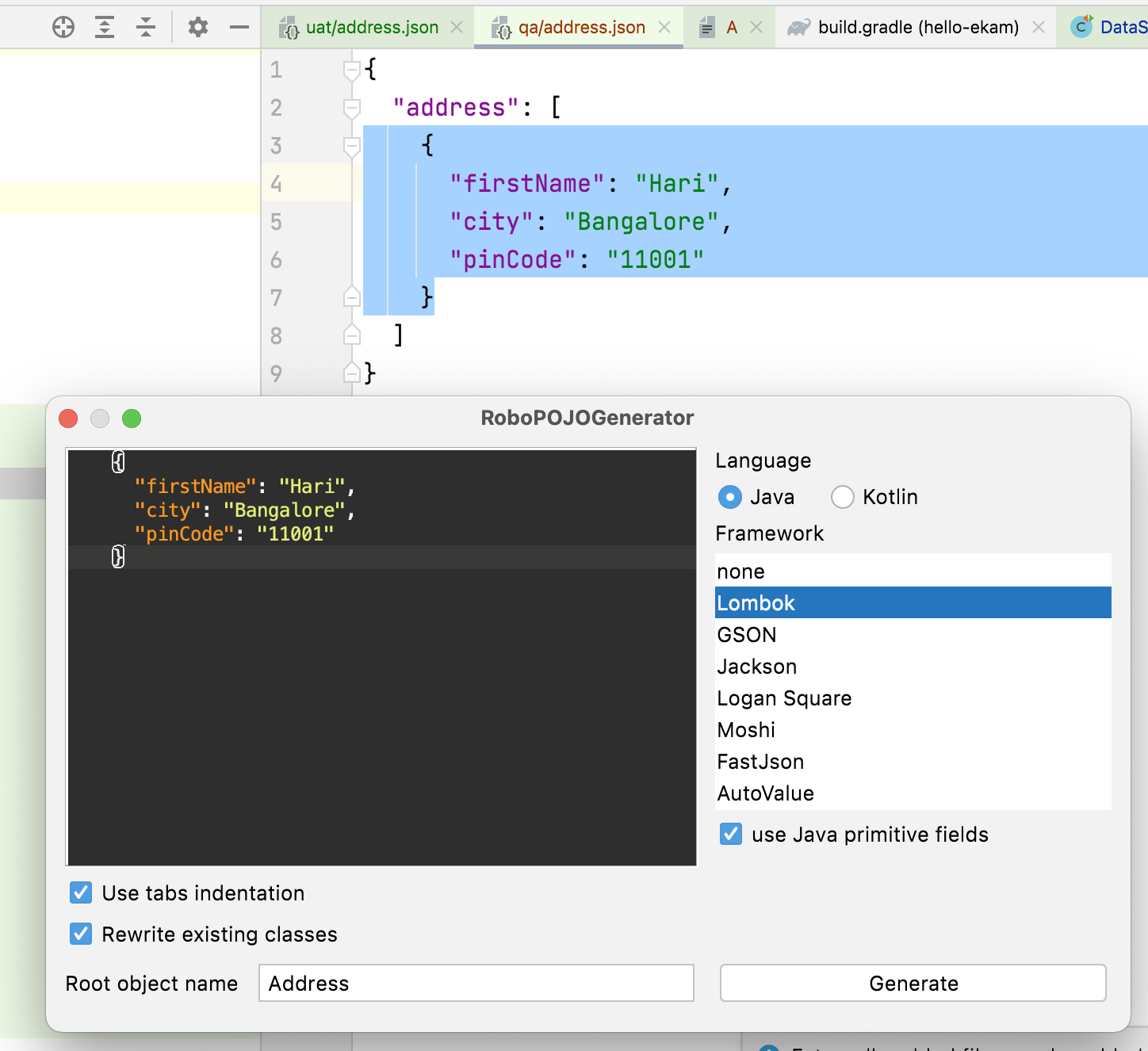
Now Address Model would get generated as below:
dataclients/address/Address.java
package dataclients.address;
import lombok.Data;
public @Data class Address{
private String firstName;
private String city;
private String pinCode;
}
Generate Data Client
Data Client is a class that reads a Model. Let us see how this is done
Right-click on dataclients/address
folder → New → Ekam Component.
In the popup choose Data Client & enter the name is AddressDataClient
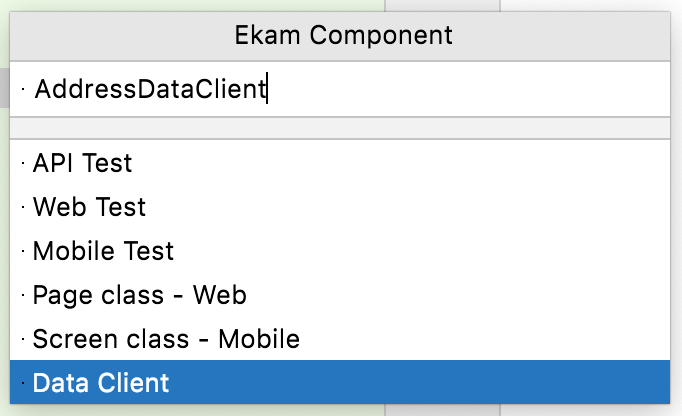
The purpose of AddressDataClient
is to read the Address
model.
Complete the AddressDataClient
by adding the method shown below to read the address object:
package dataclients.address;
import com.testvagrant.ekam.commons.data.DataSetsClient;
public class AddressDataClient extends DataSetsClient {
public Address getAddress() {
return getValue("address", Address.class);
}
}
as seen above: the key is “address” and the value would be address object
Retrieve Data
Now that we created Model and DataClient for the Model, let us see how to fetch the address object
- Inject the AddressDataClient
- Call the getValue method
package ekam.example.web;
import com.google.inject.Inject;
import com.testvagrant.ekam.testBases.testng.WebTest;
import dataclients.address.Address;
import dataclients.address.AddressDataClient;
import org.testng.annotations.Test;
public class DataSetsClientTest extends WebTest {
@Inject private AddressDataClient addressDataClient;
@Test(groups = "web")
public void shouldFetchAddress() {
Address address = addressDataClient.getAddress();
System.out.println(address.getFirstName());
}
}
Execute test
Execute test against qa environment:
./gradlew clean runWebTests -Dconfig=qa
The output would be Hari
Execute test against uat environment:
./gradlew clean runWebTests -Dconfig=uat
The output would be Puja