Logging Support
Ekam provides logging out of the box.
Logging Types
- Console logging
- File Logging
Logging levels
- Info
- Debug
Summary of Logging
Log type | Description |
---|---|
log.types: console | Logs only to console |
log.types: file | Logs only to file |
log.types: console,file | Log to both file as well as console |
Log level | Description |
---|---|
log.file.level: info | Logs only info statements to file |
log.file.level: debug | Logs both info and debug statements to file |
log.console.level: info | Logs only info statements to console |
log.console.level: debug | Logs both info and debug statements to console |
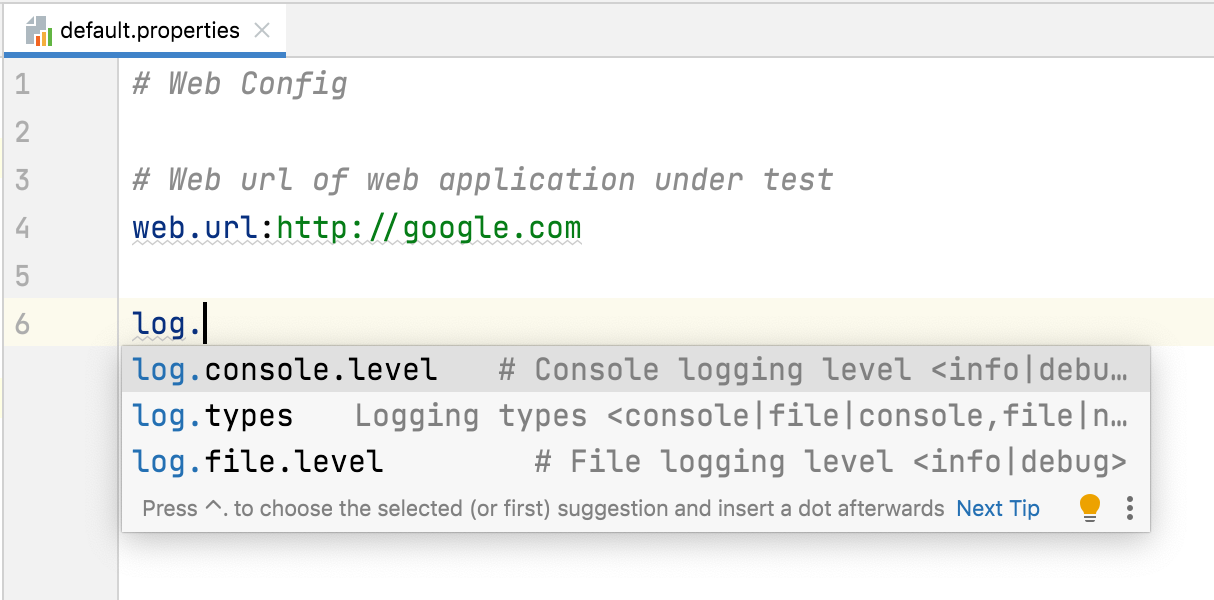
NOTE: The logging is enabled by default with below values.
log.types: console,file
log.console.level: debug
log.file.level: debug
If you wish to disable logging altogether, please add the below line to the config
log.types: none
How do we add log statements ?
Add log statements like the below examples
Debug level log
ekamLogger().debug(String.format("Searched for %s", text));
Info level log:
ekamLogger().info(String.format("Title is %s", title));
Complete example
package ekam.example.web.page;
import com.testvagrant.ekam.atoms.web.WebPage;
import com.testvagrant.ekam.reports.annotations.WebStep;
import com.testvagrant.ekam.web.annotations.WebSwitchView;
import org.openqa.selenium.By;
import static com.testvagrant.ekam.logger.EkamLogger.ekamLogger;
public class GoogleHomePage extends WebPage {
protected By searchBox = queryByName("q");
private By searchButton = query("input[value='Google Search']");
@WebSwitchView(view = GoogleHomeView.class)
@WebStep(keyword = "When", description = "I hit search button")
public GoogleHomePage search(String text) {
textbox(searchBox).setText(text);
element(searchButton).click();
ekamLogger().debug(String.format("Searched for %s", text));
return this;
}
@WebStep(keyword = "And", description = "I return Title")
public String getTitle() {
String title = driver.getTitle();
ekamLogger().info(String.format("Title is %s", title));
return title;
}
}
The logs would be available under build/logs
folder